Can a Neural Network Measure the Random Error in a Linear Series?Final layer of neural network responsible for overfittingTensorflow regression predicting 1 for all inputsMy Keras bidirectional LSTM model is giving terrible predictionsSimple prediction with KerasValueError: Error when checking target: expected dense_2 to have shape (1,) but got array with shape (0,)My Neural network in Tensorflow does a bad job in comparison to the same Neural network in KerasImplementing simple linear regression using a neural networkValidation-split of Keras fit functionValue error in Merging two different models in kerasAccuracy and Loss in MLP
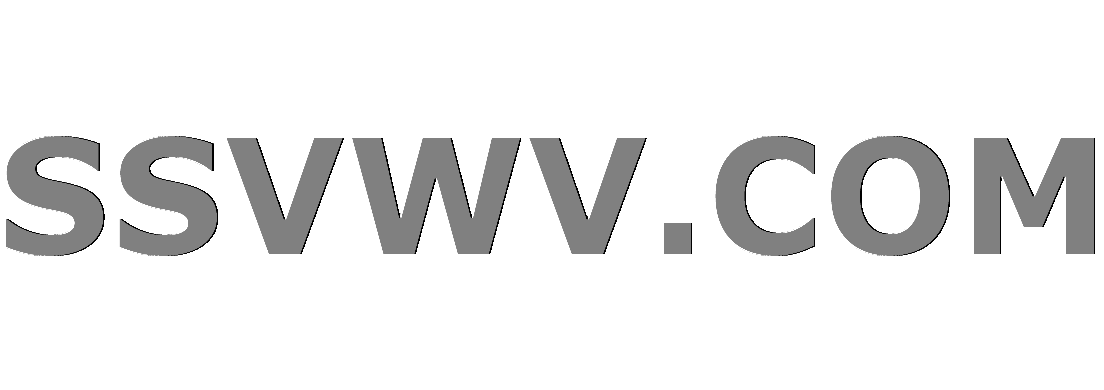
Multi tool use
Did the UK lift the requirement for registering SIM cards?
How to convince somebody that he is fit for something else, but not this job?
Why is it that I can sometimes guess the next note?
The IT department bottlenecks progress, how should I handle this?
What's the name of the logical fallacy where a debater extends a statement far beyond the original statement to make it true?
Can I turn my anal-retentiveness into a career?
Does the reader need to like the PoV character?
15% tax on $7.5k earnings. Is that right?
How do I fix the group tension caused by my character stealing and possibly killing without provocation?
Does grappling negate Mirror Image?
Change the color of a single dot in `ddot` symbol
Taxes on Dividends in a Roth IRA
"It doesn't matter" or "it won't matter"?
"before" and "want" for the same systemd service?
I found an audio circuit and I built it just fine, but I find it a bit too quiet. How do I amplify the output so that it is a bit louder?
Will number of steps recorded on FitBit/any fitness tracker add up distance in PokemonGo?
Does "he squandered his car on drink" sound natural?
Permission on Database
Do we have to expect a queue for the shuttle from Watford Junction to Harry Potter Studio?
Are cause and effect the same as in our Universe in a non-relativistic, Newtonian Universe in which the speed of light is infinite?
When were female captains banned from Starfleet?
What is the English pronunciation of "pain au chocolat"?
Does Doodling or Improvising on the Piano Have Any Benefits?
What is the difference between lands and mana?
Can a Neural Network Measure the Random Error in a Linear Series?
Final layer of neural network responsible for overfittingTensorflow regression predicting 1 for all inputsMy Keras bidirectional LSTM model is giving terrible predictionsSimple prediction with KerasValueError: Error when checking target: expected dense_2 to have shape (1,) but got array with shape (0,)My Neural network in Tensorflow does a bad job in comparison to the same Neural network in KerasImplementing simple linear regression using a neural networkValidation-split of Keras fit functionValue error in Merging two different models in kerasAccuracy and Loss in MLP
$begingroup$
I have been trying to develop a neural network to measure the error in a linear series. What I would like the model to do is infer a linear regression line and then measure the mean absolute error around that line.
I have tried a number of neural network model configurations, including recurrent configurations, but the network learns a weak relationship and then overfits. I have also tried L1 and L2 regularization but neither work.
Any thoughts? Thanks!
Below is the code I am using to simulate the data and a fit sample model:
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
X = np.array(()).reshape(0, 50)
Y = np.array(()).reshape(0, 1)
for _ in range(500):
i = np.random.randint(100, 110) # Intercept.
s = np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, 50) # Error.
X_i = np.round(i + (s * np.arange(0, 50)) + e, 2).reshape(1, 50)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 400
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(512, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(512, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = None))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 100, verbose = False,
validation_data = (X_valid, Y_valid))
## Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.plot(epochs_fnn, loss_fnn, 'black', label = 'Training Loss')
plt.plot(epochs_fnn, val_loss_fnn, 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
plt.show()
UPDATE:
## Predict.
Y_train_fnn = model_fnn.predict(X_train)
Y_valid_fnn = model_fnn.predict(X_valid)
## Evaluate predictions with training data.
plt.scatter(Y_train, Y_train_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
## Evaluate predictions with training data.
plt.scatter(Y_valid, Y_valid_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
python neural-network keras linear-regression
$endgroup$
add a comment |
$begingroup$
I have been trying to develop a neural network to measure the error in a linear series. What I would like the model to do is infer a linear regression line and then measure the mean absolute error around that line.
I have tried a number of neural network model configurations, including recurrent configurations, but the network learns a weak relationship and then overfits. I have also tried L1 and L2 regularization but neither work.
Any thoughts? Thanks!
Below is the code I am using to simulate the data and a fit sample model:
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
X = np.array(()).reshape(0, 50)
Y = np.array(()).reshape(0, 1)
for _ in range(500):
i = np.random.randint(100, 110) # Intercept.
s = np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, 50) # Error.
X_i = np.round(i + (s * np.arange(0, 50)) + e, 2).reshape(1, 50)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 400
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(512, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(512, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = None))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 100, verbose = False,
validation_data = (X_valid, Y_valid))
## Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.plot(epochs_fnn, loss_fnn, 'black', label = 'Training Loss')
plt.plot(epochs_fnn, val_loss_fnn, 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
plt.show()
UPDATE:
## Predict.
Y_train_fnn = model_fnn.predict(X_train)
Y_valid_fnn = model_fnn.predict(X_valid)
## Evaluate predictions with training data.
plt.scatter(Y_train, Y_train_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
## Evaluate predictions with training data.
plt.scatter(Y_valid, Y_valid_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
python neural-network keras linear-regression
$endgroup$
add a comment |
$begingroup$
I have been trying to develop a neural network to measure the error in a linear series. What I would like the model to do is infer a linear regression line and then measure the mean absolute error around that line.
I have tried a number of neural network model configurations, including recurrent configurations, but the network learns a weak relationship and then overfits. I have also tried L1 and L2 regularization but neither work.
Any thoughts? Thanks!
Below is the code I am using to simulate the data and a fit sample model:
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
X = np.array(()).reshape(0, 50)
Y = np.array(()).reshape(0, 1)
for _ in range(500):
i = np.random.randint(100, 110) # Intercept.
s = np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, 50) # Error.
X_i = np.round(i + (s * np.arange(0, 50)) + e, 2).reshape(1, 50)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 400
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(512, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(512, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = None))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 100, verbose = False,
validation_data = (X_valid, Y_valid))
## Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.plot(epochs_fnn, loss_fnn, 'black', label = 'Training Loss')
plt.plot(epochs_fnn, val_loss_fnn, 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
plt.show()
UPDATE:
## Predict.
Y_train_fnn = model_fnn.predict(X_train)
Y_valid_fnn = model_fnn.predict(X_valid)
## Evaluate predictions with training data.
plt.scatter(Y_train, Y_train_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
## Evaluate predictions with training data.
plt.scatter(Y_valid, Y_valid_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
python neural-network keras linear-regression
$endgroup$
I have been trying to develop a neural network to measure the error in a linear series. What I would like the model to do is infer a linear regression line and then measure the mean absolute error around that line.
I have tried a number of neural network model configurations, including recurrent configurations, but the network learns a weak relationship and then overfits. I have also tried L1 and L2 regularization but neither work.
Any thoughts? Thanks!
Below is the code I am using to simulate the data and a fit sample model:
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
X = np.array(()).reshape(0, 50)
Y = np.array(()).reshape(0, 1)
for _ in range(500):
i = np.random.randint(100, 110) # Intercept.
s = np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, 50) # Error.
X_i = np.round(i + (s * np.arange(0, 50)) + e, 2).reshape(1, 50)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 400
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(512, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(512, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = None))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 100, verbose = False,
validation_data = (X_valid, Y_valid))
## Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.plot(epochs_fnn, loss_fnn, 'black', label = 'Training Loss')
plt.plot(epochs_fnn, val_loss_fnn, 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
plt.show()
UPDATE:
## Predict.
Y_train_fnn = model_fnn.predict(X_train)
Y_valid_fnn = model_fnn.predict(X_valid)
## Evaluate predictions with training data.
plt.scatter(Y_train, Y_train_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
## Evaluate predictions with training data.
plt.scatter(Y_valid, Y_valid_fnn)
plt.xlabel("Actual")
plt.ylabel("Predicted")
python neural-network keras linear-regression
python neural-network keras linear-regression
edited Mar 18 at 21:57
from keras import michael
asked Mar 18 at 18:02


from keras import michaelfrom keras import michael
29810
29810
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
This problem is naturally hard. The underlying function that we try to learn is
$$mathbfX=i+s+mathbfe rightarrow Y=left | mathbfX - i - s right |_1 = left | mathbfe right |_1=sum_d|e_d|$$
where $i$ and $s$ are unknown random variables. For large $i$ and $s$, $left | mathbfe right |_1$ is naturally hard to recover from $mathbfX$. I found a working example (training error almost zero) by setting the intercept $i$ and slope $s$ to zero!, drastically shrinking the network size to work better with a small sample size (800), and increased the number of epochs to 800, which was crucial. Also, (true value, error) is plotted at the end for training data.
You can work up from this point to see the effect of increasing $i$ and $s$ on performance.
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
dimension = 50
X = np.array(()).reshape(0, dimension)
Y = np.array(()).reshape(0, 1)
for _ in range(1000):
i = 0 # np.random.randint(100, 110) # Intercept.
s = 0 # np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, dimension) # Error.
X_i = np.round(i + (s * np.arange(0, dimension)) + e, 2).reshape(1, dimension)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 800
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(dimension, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(dimension, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = 'linear'))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 800, verbose = True,
validation_data = (X_valid, Y_valid))
# Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.figure(1)
offset = 5
plt.plot(epochs_fnn[offset:], loss_fnn[offset:], 'black', label = 'Training Loss')
plt.plot(epochs_fnn[offset:], val_loss_fnn[offset:], 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
## Predict.
plt.figure(2)
Y_train_fnn = model_fnn.predict(X_train)
## Evaluate predictions with training data.
sorted_index = Y_train.argsort(axis=0)
Y_train_sorted = np.reshape(Y_train[sorted_index], (-1, 1))
Y_train_fnn_sorted = np.reshape(Y_train_fnn[sorted_index], (-1, 1))
plt.plot(Y_train_sorted, Y_train_sorted - Y_train_fnn_sorted)
plt.xlabel("Y(true) train")
plt.ylabel("Y(true) - Y(predicted) train")
plt.show()
$endgroup$
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
return StackExchange.using("mathjaxEditing", function ()
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix)
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["$", "$"], ["\\(","\\)"]]);
);
);
, "mathjax-editing");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "557"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdatascience.stackexchange.com%2fquestions%2f47550%2fcan-a-neural-network-measure-the-random-error-in-a-linear-series%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
This problem is naturally hard. The underlying function that we try to learn is
$$mathbfX=i+s+mathbfe rightarrow Y=left | mathbfX - i - s right |_1 = left | mathbfe right |_1=sum_d|e_d|$$
where $i$ and $s$ are unknown random variables. For large $i$ and $s$, $left | mathbfe right |_1$ is naturally hard to recover from $mathbfX$. I found a working example (training error almost zero) by setting the intercept $i$ and slope $s$ to zero!, drastically shrinking the network size to work better with a small sample size (800), and increased the number of epochs to 800, which was crucial. Also, (true value, error) is plotted at the end for training data.
You can work up from this point to see the effect of increasing $i$ and $s$ on performance.
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
dimension = 50
X = np.array(()).reshape(0, dimension)
Y = np.array(()).reshape(0, 1)
for _ in range(1000):
i = 0 # np.random.randint(100, 110) # Intercept.
s = 0 # np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, dimension) # Error.
X_i = np.round(i + (s * np.arange(0, dimension)) + e, 2).reshape(1, dimension)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 800
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(dimension, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(dimension, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = 'linear'))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 800, verbose = True,
validation_data = (X_valid, Y_valid))
# Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.figure(1)
offset = 5
plt.plot(epochs_fnn[offset:], loss_fnn[offset:], 'black', label = 'Training Loss')
plt.plot(epochs_fnn[offset:], val_loss_fnn[offset:], 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
## Predict.
plt.figure(2)
Y_train_fnn = model_fnn.predict(X_train)
## Evaluate predictions with training data.
sorted_index = Y_train.argsort(axis=0)
Y_train_sorted = np.reshape(Y_train[sorted_index], (-1, 1))
Y_train_fnn_sorted = np.reshape(Y_train_fnn[sorted_index], (-1, 1))
plt.plot(Y_train_sorted, Y_train_sorted - Y_train_fnn_sorted)
plt.xlabel("Y(true) train")
plt.ylabel("Y(true) - Y(predicted) train")
plt.show()
$endgroup$
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
add a comment |
$begingroup$
This problem is naturally hard. The underlying function that we try to learn is
$$mathbfX=i+s+mathbfe rightarrow Y=left | mathbfX - i - s right |_1 = left | mathbfe right |_1=sum_d|e_d|$$
where $i$ and $s$ are unknown random variables. For large $i$ and $s$, $left | mathbfe right |_1$ is naturally hard to recover from $mathbfX$. I found a working example (training error almost zero) by setting the intercept $i$ and slope $s$ to zero!, drastically shrinking the network size to work better with a small sample size (800), and increased the number of epochs to 800, which was crucial. Also, (true value, error) is plotted at the end for training data.
You can work up from this point to see the effect of increasing $i$ and $s$ on performance.
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
dimension = 50
X = np.array(()).reshape(0, dimension)
Y = np.array(()).reshape(0, 1)
for _ in range(1000):
i = 0 # np.random.randint(100, 110) # Intercept.
s = 0 # np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, dimension) # Error.
X_i = np.round(i + (s * np.arange(0, dimension)) + e, 2).reshape(1, dimension)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 800
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(dimension, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(dimension, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = 'linear'))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 800, verbose = True,
validation_data = (X_valid, Y_valid))
# Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.figure(1)
offset = 5
plt.plot(epochs_fnn[offset:], loss_fnn[offset:], 'black', label = 'Training Loss')
plt.plot(epochs_fnn[offset:], val_loss_fnn[offset:], 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
## Predict.
plt.figure(2)
Y_train_fnn = model_fnn.predict(X_train)
## Evaluate predictions with training data.
sorted_index = Y_train.argsort(axis=0)
Y_train_sorted = np.reshape(Y_train[sorted_index], (-1, 1))
Y_train_fnn_sorted = np.reshape(Y_train_fnn[sorted_index], (-1, 1))
plt.plot(Y_train_sorted, Y_train_sorted - Y_train_fnn_sorted)
plt.xlabel("Y(true) train")
plt.ylabel("Y(true) - Y(predicted) train")
plt.show()
$endgroup$
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
add a comment |
$begingroup$
This problem is naturally hard. The underlying function that we try to learn is
$$mathbfX=i+s+mathbfe rightarrow Y=left | mathbfX - i - s right |_1 = left | mathbfe right |_1=sum_d|e_d|$$
where $i$ and $s$ are unknown random variables. For large $i$ and $s$, $left | mathbfe right |_1$ is naturally hard to recover from $mathbfX$. I found a working example (training error almost zero) by setting the intercept $i$ and slope $s$ to zero!, drastically shrinking the network size to work better with a small sample size (800), and increased the number of epochs to 800, which was crucial. Also, (true value, error) is plotted at the end for training data.
You can work up from this point to see the effect of increasing $i$ and $s$ on performance.
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
dimension = 50
X = np.array(()).reshape(0, dimension)
Y = np.array(()).reshape(0, 1)
for _ in range(1000):
i = 0 # np.random.randint(100, 110) # Intercept.
s = 0 # np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, dimension) # Error.
X_i = np.round(i + (s * np.arange(0, dimension)) + e, 2).reshape(1, dimension)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 800
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(dimension, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(dimension, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = 'linear'))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 800, verbose = True,
validation_data = (X_valid, Y_valid))
# Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.figure(1)
offset = 5
plt.plot(epochs_fnn[offset:], loss_fnn[offset:], 'black', label = 'Training Loss')
plt.plot(epochs_fnn[offset:], val_loss_fnn[offset:], 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
## Predict.
plt.figure(2)
Y_train_fnn = model_fnn.predict(X_train)
## Evaluate predictions with training data.
sorted_index = Y_train.argsort(axis=0)
Y_train_sorted = np.reshape(Y_train[sorted_index], (-1, 1))
Y_train_fnn_sorted = np.reshape(Y_train_fnn[sorted_index], (-1, 1))
plt.plot(Y_train_sorted, Y_train_sorted - Y_train_fnn_sorted)
plt.xlabel("Y(true) train")
plt.ylabel("Y(true) - Y(predicted) train")
plt.show()
$endgroup$
This problem is naturally hard. The underlying function that we try to learn is
$$mathbfX=i+s+mathbfe rightarrow Y=left | mathbfX - i - s right |_1 = left | mathbfe right |_1=sum_d|e_d|$$
where $i$ and $s$ are unknown random variables. For large $i$ and $s$, $left | mathbfe right |_1$ is naturally hard to recover from $mathbfX$. I found a working example (training error almost zero) by setting the intercept $i$ and slope $s$ to zero!, drastically shrinking the network size to work better with a small sample size (800), and increased the number of epochs to 800, which was crucial. Also, (true value, error) is plotted at the end for training data.
You can work up from this point to see the effect of increasing $i$ and $s$ on performance.
import numpy as np, matplotlib.pyplot as plt
from keras import layers
from keras.models import Sequential
from keras.optimizers import Adam
from keras.backend import clear_session
## Simulate the data:
np.random.seed(20190318)
dimension = 50
X = np.array(()).reshape(0, dimension)
Y = np.array(()).reshape(0, 1)
for _ in range(1000):
i = 0 # np.random.randint(100, 110) # Intercept.
s = 0 # np.random.randint(1, 10) # Slope.
e = np.random.normal(0, 25, dimension) # Error.
X_i = np.round(i + (s * np.arange(0, dimension)) + e, 2).reshape(1, dimension)
Y_i = np.sum(np.abs(e)).reshape(1, 1)
X = np.concatenate((X, X_i), axis = 0)
Y = np.concatenate((Y, Y_i), axis = 0)
## Training and validation data:
split = 800
X_train = X[:split, :-1]
Y_train = Y[:split, -1:]
X_valid = X[split:, :-1]
Y_valid = Y[split:, -1:]
print(X_train.shape)
print(Y_train.shape)
print()
print(X_valid.shape)
print(Y_valid.shape)
## Graph of one of the series:
plt.plot(X_train[0])
## Sample model (takes about a minute to run):
clear_session()
model_fnn = Sequential()
model_fnn.add(layers.Dense(dimension, activation = 'relu', input_shape = (X_train.shape[1],)))
model_fnn.add(layers.Dense(dimension, activation = 'relu'))
model_fnn.add(layers.Dense( 1, activation = 'linear'))
# Compile model.
model_fnn.compile(optimizer = Adam(lr = 1e-4), loss = 'mse')
# Fit model.
history_fnn = model_fnn.fit(X_train, Y_train, batch_size = 32, epochs = 800, verbose = True,
validation_data = (X_valid, Y_valid))
# Sample model learning curves:
loss_fnn = history_fnn.history['loss']
val_loss_fnn = history_fnn.history['val_loss']
epochs_fnn = range(1, len(loss_fnn) + 1)
plt.figure(1)
offset = 5
plt.plot(epochs_fnn[offset:], loss_fnn[offset:], 'black', label = 'Training Loss')
plt.plot(epochs_fnn[offset:], val_loss_fnn[offset:], 'red', label = 'Validation Loss')
plt.title('FNN: Training and Validation Loss')
plt.legend()
## Predict.
plt.figure(2)
Y_train_fnn = model_fnn.predict(X_train)
## Evaluate predictions with training data.
sorted_index = Y_train.argsort(axis=0)
Y_train_sorted = np.reshape(Y_train[sorted_index], (-1, 1))
Y_train_fnn_sorted = np.reshape(Y_train_fnn[sorted_index], (-1, 1))
plt.plot(Y_train_sorted, Y_train_sorted - Y_train_fnn_sorted)
plt.xlabel("Y(true) train")
plt.ylabel("Y(true) - Y(predicted) train")
plt.show()
edited 2 days ago
answered Mar 18 at 19:10
EsmailianEsmailian
1,651114
1,651114
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
add a comment |
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Is there a way to make the problem easier to solve? As humans, we know we can fit a regression line and sum the differences. Can a neural network learn to do this?
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Let us continue this discussion in chat.
$endgroup$
– from keras import michael
2 days ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
$begingroup$
Working with @esmailian, we solved it by first creating a network to infer predicted values based on a regression line, then a second network to measure the MAE between the predicted and actual values. Of note is that many more observations (20,000) were required because the network struggled to learn the MAE function with 500 or 800 observations.
$endgroup$
– from keras import michael
8 hours ago
add a comment |
Thanks for contributing an answer to Data Science Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fdatascience.stackexchange.com%2fquestions%2f47550%2fcan-a-neural-network-measure-the-random-error-in-a-linear-series%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HJ8qIe,kzp